Introduction to JavaScript: Control Flow

Often times we want our program to act differently based on the information supplied to it. This is known as control flow, and is an integral part of software development.
At the end of this article, you should be able to:
- understand and be able to use different operators.
- write control flow using if/else statements.
- write a basic for loop.
Requirements
Learn
Learn to understand and be able to use different operators.
Overview
Operators allow software developers to compare variables and enable programs to make decisions about control flow. In order to understand control flow, we must first understand some basic operators.
Undefined and Null
There are a couple of JavaScript objects that don’t really fit into any type. Those are the values undefined
and null
. You will get undefined
when you are looking for something that does not exist like a variable that does not have a value yet. undefined
simply means what you are asking for does not exist.

null
is an object that we, the developers, set when we want to tell other developers that the item they are looking for exists, but there is no value associated with it. While undefined
is set by the JavaScript language, null
is set by the developer. If you ever receive null
, know that another developer has set that value to null

One last thing to note, neither undefined
nor null
are strings. They are written just as they are with no quotes around them, like a Boolean.
Truthiness
The Boolean values, true
and false
. When using an if
statement or other statements that expect a Boolean value (such as the !
, NOT), and the expression given is not a Boolean value, JavaScript will do something called type coercion and transform whatever it is given into a Boolean value. This is known as truthy and falsey. Every data type has a truthiness to it. Here are some examples:
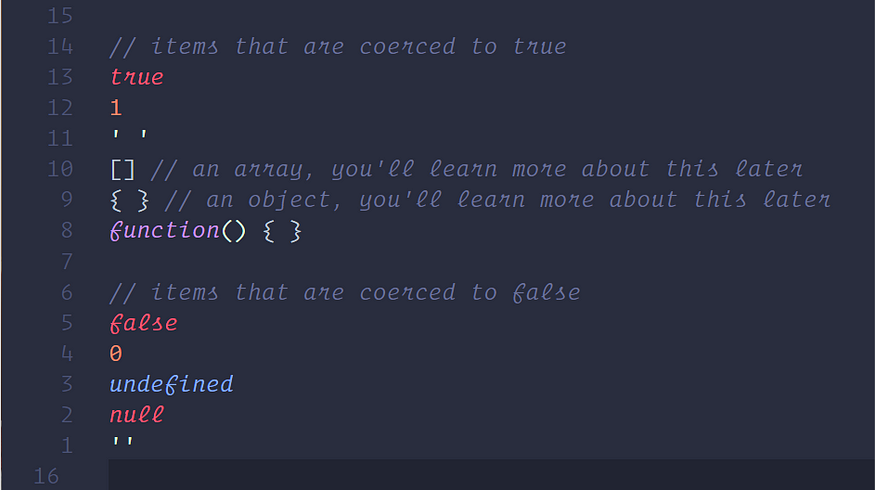
Comparison Operators
Comparison operators (>
>=
<
<=
===
!==
). These operators work just as they would in a math class: greater than, less than, and etc. We use these operators to evaluate two expressions. As the computer runs the code, the operator will return either a true
(if the statement is true) or a false
(if the statement is not true).

The “triple equals” sign ( ===
) must not be confused with a single equal sign (which indicates assigning a value to a variable). The triple equal will compare everything about the two items, including type, and return if they are exactly equal or not: (Something to note: there is a “double equals” ( ==
) sign which will compare two items, but it will NOT take into account their types (1 == '1' // true
). Due to this, it is considered bad practice to use the double equal sign. We would like to see you always using the triple equal sign, and you will always see us using it.)

The last comparison operator we would like to introduce you to has two parts to it.
First is the “NOT” (!
). When you see this, it will mean that we are asking the opposite of the expression (we will revisit the NOT operator later in this lesson).
With that in mind, we can introduce the “not equals” ( !==
) sign. This will return true if the items are NOT equal to each other, in any way. This, like the triple equal sign, takes type into account.

Logical Operators
We can also combine two equality expressions and ask if either of them are true, both of them are true, or neither of them is true. To do this we will use Logical Operators.
&&
The first logical operator we will look at is the “AND” operator. It is written with two ampersands (&&
). This will evaluate both expressions and will return true if BOTH expressions are true. If one (or both) of them is false, then this operator will return false:

||
The next is the “OR” operator. It is written with two vertical bars (||
). It will determine if one of the expressions is true
. It will return true
if one (or both) of the expressions is true
. It will return false
if BOTH expressions are false
:

!
The last logical operator is the “NOT” operator. It is written as a single exclamation mark (!
). We saw this operator earlier when determining equality (!==
). As before, the NOT operator will return the opposite Boolean value of what is passed to it:

Notes About Logical Operators
A couple things to note about logical operators.
- The expressions are evaluated in order, and the computer will skip any redundant expressions. In an
&&
statement, if the first expression isfalse
, the second expression will not be evaluated because BOTH expressions need to betrue
. Same for the||
statement. If the first expression istrue
, the second will not be evaluated because there only needs to be onetrue
statement to fulfill the requirements of the operator. - Use parentheses. As we saw in the second
!
operator example, we used parentheses to evaluate what was inside of the parentheses FIRST, then applied the!
operator. We can wrap ANY expression in parentheses and it will be evaluated before evaluating the expression as a whole.
Learn
Learn to write control flow using if/else statements.
Overview
We touched on control flow in our last lesson, now we will introduce some more complexity. Things aren’t always black or white. Sometimes there is a third (or fourth, or hundredth, etc.) option.
Control Flow (continued)
In the last lesson, we learned about the if
operator. We can use if
to check and see if an expression is true
. If it is, run some code. If it is not, skip the code and keep running the program.

To add on to if
, we can also use the else if
and else
statements. These statements must be used with if
and must come after it. These statements will be evaluated if the initial if
returns false
. We can think of the else if
as another if
statement that has been chained (we can have as many else if statements we want). Only one if
or else if
statement code block will be run. If at any time a statement returns true
, that code will be run and the rest will be skipped:
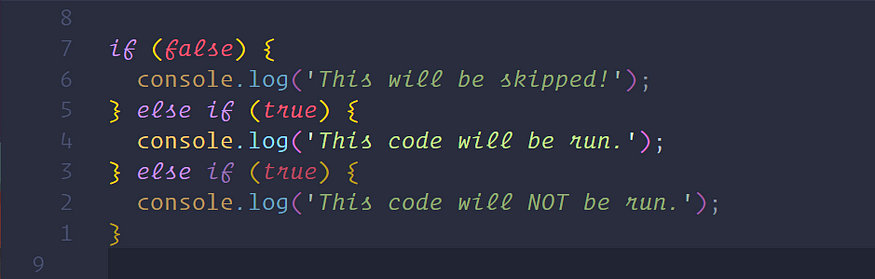
The else
statement will always come at the end of an if-else if
chain, and will act as a default. If none of the expressions returned true
, the else
code block will be run no matter what. If any of the previous if
or else if
expressions are true
, the else
statement code block will not be run.

Why use
else if
?
You may be thinking to yourself, “Why not just use a bunch of different if
statements? Why use else if
? That is a valid question, and sometimes that is the right approach to take. It is up to you to decide which is the right approach. Remember, when using an if/else if
block, only ONE of the statements will be run, even if there are multiple statements that are true. The same can not be said for multiple if
statements; they will run if true no matter what. Take this example:

In the above example only older than 20!
will be logged even though both the if
statement and else if
statement were true.

In the above example the first and second if statements would console log because both of them are true.
As you can see, it will be up to you to decide if you want to have multiple actions based on your data or just one action.
Learn
Learn to write a basic for loop.
Overview
Loops are how software ‘runs’. Understand basic loops and the native for
loop implementation in JavaScript.
Loops
Most software runs on loops, evaluating expressions over and over again until it either returns what we are looking for, or stops after a certain time. JavaScript has two looping expressions built into it and today we will look at the first one, the “for” loop.
for Loops
for
loops have a unique syntax, similar to the if
statement, but slightly more complex. First, we have the for
keyword, followed by parentheses, and then open and close braces. Within the parentheses, we will need three things. First, we must declare a variable. This is what the loop will be looping over. Then, we will have a conditional expression. The loop will continue happening until this statement is false
. Third, we will increment our variable. All three of these statements are separated by a semicolon.

In this example, we see that we initially set our counter
variable to 0. The loop will run and each time it gets to the end, it will increase the counter by one. The for loop will then evaluate the conditional expression. If it is true
, it will run again, but if it is false
it will stop running.
The ++ operator
We saw in the last two examples the ++
operator. This is JavaScript shorthand for “Set the value of the variable to its current value plus one”. There are a few more of these variable math/assignment shorthand expressions. We will visit them in upcoming lessons.
Infinite Loops.
It is possible to get your loop stuck in what we call an “Infinite Loop”. You must make sure there is a way for the loop to end. Take for example this loop:

Because our conditional expression will ALWAYS be true
(i
will never be less than 0) this loop will essentially run forever. This will break your program, and may crash your web browser, or computer.
Thank you for useful content..
ReplyDelete